반응형
프로젝트 생성
먼저, 다음과 같은 환경으로 프로젝트를 구성합니다.
intellij community 버전이기 때문에 spring boot 공식 홈페이지에서 만들었습니다.
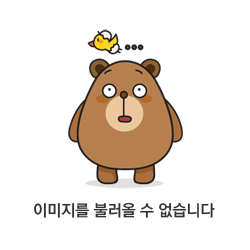
프로젝트를 생성한 후 build.gradle의 dependencies를 확인하면 다음과 같습니다.
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
implementation 'org.springframework.boot:spring-boot-starter-web'
compileOnly 'org.projectlombok:lombok'
runtimeOnly 'com.h2database:h2'
annotationProcessor 'org.projectlombok:lombok'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
h2 Database 설정
application.properties에 다음과 같이 설정합니다.
# set h2 properties
spring.h2.console.enabled=true
# 임의 명칭 생성 여부 (true-랜덤 생성, false-명칭 고정)
spring.datasource.generate-unique-name=false
# /h2-console이 기본값이기 때문에 생략 가능
spring.h2.console.path=/h2-console
spring.datasouce.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
#jpa
spring.jpa.hibernate.ddl-auto=create
spring.jpa.generate-ddl=true
spring.jpa.show-sql=true
spring.jpa.database=h2
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
#logging
logging.level.org.springframework.web=DEBUG
logging.level.org.hibernate.sql=DEBUG
logging.level.jpa=DEBUG
JPA에 필요한 클래스 파일 생성
Entity Class
- Database 테이블명이 TB_USER라면, TbUser라는 클래스를 생성하면 자동으로 mapping 됩니다.
package com.example.demo.entity;
import lombok.Getter;
import lombok.Setter;
import javax.persistence.*;
@Entity
@Table
@Getter
@Setter
public class TbUser {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long userCode;
@Column(length=50, nullable = false)
private String userId;
@Column(length=50, nullable = false)
private String userName;
}
JpaRepository
- JpaRepository를 상속받은 TbUserRepository 인터페이스를 생성합니다.
- 간혹 인터넷을 검색해보면 @Repository 어노테이션을 사용한 예제를 볼 수 있는데, 없어도 상관없습니다.
import com.example.demo.entity.TbUser;
import org.springframework.data.jpa.repository.JpaRepository;
public interface TbUserRepository extends JpaRepository<TbUser, Long> {
}
parameter를 받을 클래스를 생성
package com.example.demo;
import lombok.Getter;
import lombok.Setter;
@Getter
@Setter
public class UserParam {
private String userId;
private String userName;
}
Controller
API 테스트를 진행할 Controller를 생성합니다.
테스트이기 때문에 사용자 추가와 사용자 정보만 생성했습니다.
package com.example.demo;
import com.example.demo.entity.TbUser;
import com.example.demo.repository.TbUserRepository;
import java.util.Optional;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequiredArgsConstructor
@Slf4j
public class UserController {
private final TbUserRepository userRepository;
@PostMapping("/users")
public ResponseEntity<Long> createUser(@RequestBody UserParam param) {
log.info(param.toString());
TbUser user = new TbUser();
user.setUserId(param.getUserId());
user.setUserName(param.getUserName());
userRepository.save(user);
return new ResponseEntity<Long>(user.getUserCode(), HttpStatus.CREATED);
}
@GetMapping("/users/{id}")
public ResponseEntity<TbUser> userList(@PathVariable Long id) {
Optional<TbUser> user = userRepository.findById(id);
if(user.isPresent()){
return new ResponseEntity<TbUser>(user.get(), HttpStatus.OK);
}
else{
return new ResponseEntity<TbUser>(HttpStatus.NOT_FOUND);
}
}
}
테스트 클래스 생성
package com.example.demo;
import static org.junit.jupiter.api.Assertions.*;
import org.json.JSONException;
import org.json.JSONObject;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.mock.web.MockHttpServletResponse;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
@AutoConfigureMockMvc
@SpringBootTest
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void createUser() throws Exception {
String url = "/users";
JSONObject obj = new JSONObject();
obj.put("userId", "hong");
obj.put("userName", "hone gil dong");
System.out.println("obj:" + obj.toString());
mockMvc.perform(MockMvcRequestBuilders.post(url)
.content(obj.toString())
.contentType(MediaType.APPLICATION_JSON))
.andExpect(result -> {
MockHttpServletResponse response = result.getResponse();
String userCode = response.getContentAsString();
System.out.println("userCode:" + userCode);
// 사용자 등록 성공 여부 확인.
Assertions.assertEquals(HttpStatus.CREATED.value(), response.getStatus());
// 사용자 정보 구하기 확인
mockMvc.perform(MockMvcRequestBuilders.get(url + "/" + userCode)
.content(obj.toString())
.contentType(MediaType.APPLICATION_JSON))
.andExpect(result2 -> {
MockHttpServletResponse response2 = result2.getResponse();
String userCode2 = response2.getContentAsString();
System.out.println("userCode2:" + userCode2);
System.out.println(response2.getStatus());
// 사용자 정보구하기 성공 여부 확인.
Assertions.assertEquals(HttpStatus.OK.value(), response2.getStatus());
});
});
}
}
728x90
반응형
'웹 개발' 카테고리의 다른 글
[CI] jenkins 설치 방법 for windows (0) | 2022.03.22 |
---|---|
junit를 사용한 단위 테스트에서 autowired가 동작하지 않을 경우 해결 방법 (0) | 2022.03.20 |
thymeleaf vs jsp (0) | 2022.03.13 |
spring boot의 application.properties에서 @Scheduled의 cron값을 설정하는 방법 (0) | 2022.03.13 |
html파일을 local에서 실행할 때 CORS 에러가 발생하는 이유 (0) | 2022.03.02 |